Write and run SQL and Python code in a Snowflake Notebook¶
When you create a notebook, three example cells are displayed. You can modify those cells or add new ones.
Write SQL code in a notebook¶
To start writing SQL code in a notebook, add a SQL cell.
To add a cell, either use a keyboard shortcut and select SQL, or select + SQL.
When writing SQL in a SQL cell, consider the following:
SQL cells must only contain a single statement per cell, and must be valid Snowflake SQL.
The single SQL statement can include CTEs.
If you need to reference the results of a query in another cell, see Reference results and chain cells in a notebook.
You cannot partially run a cell.
For other limitations, see Considerations for writing notebooks and adding cells.
Write Snowpark Python code in a notebook¶
To start writing Python code in a notebook, add a Python cell.
To add a Python cell, either use a keyboard shortcut or select + Python.
Snowflake Notebooks support the same Python version supported by Snowpark, Python 3.8.
When you create a notebook, the first Python cell imports the streamlit
library and creates a Snowpark session. The Streamlit
library is required to run a Snowflake Notebook and display visualizations.
A Snowflake Notebook creates a Snowpark session, so you can use most of the methods available in a Snowpark Session class. However, because a notebook runs inside Snowflake rather than in your local development environment, you cannot use the following methods:
session.add_import
session.add_packages
session.add_requirements
When you run a Python cell, output from the following functions is displayed in the results:
Functions that write to the console, such as
print()
.Functions that print a DataFrame, such as the
show
method of the DataFrame class in Snowpark Python.
You can import Python libraries to use in a notebook. See Import packages to use in notebooks for details.
Import packages to use in notebooks¶
Snowflake Notebooks include Streamlit and the third-party packages listed in the Snowflake Anaconda channel.
After your organization administrator accepts the terms, you can import libraries to use in Snowflake Notebooks:
Sign in to Snowsight.
Select Notebooks.
Select a specific notebook for which you want to install Python packages.
(Optional) Select Packages.
Search for packages listed in the Snowflake Anaconda channel, such as numpy, pandas, requests, and urllib3.
Select a package to install it for use in your notebook, and optionally change the default package version in the list of Installed Packages.
Packages installed by you appear under Installed Packages.
To use the previously installed libraries, add
import
statements to the code in a Python cell.For example, to use the previously installed scikit-learn package, add an
import
statement for that package at the beginning of your code:import scikit-learn
참고
Packages that you add to a notebook are available only to that notebook. If you want to use the same package in a different Snowflake Notebook, you must use this procedure to add the package to that notebook.
Write Markdown in a notebook¶
To write Markdown in your notebook, add a Markdown cell:
Use a keyboard shortcut and select Markdown, or select + Markdown.
Select the Edit markdown pencil icon, and start writing Markdown.
You can type valid Markdown to format a text cell. As you type, the formatted text appears below the Markdown syntax.
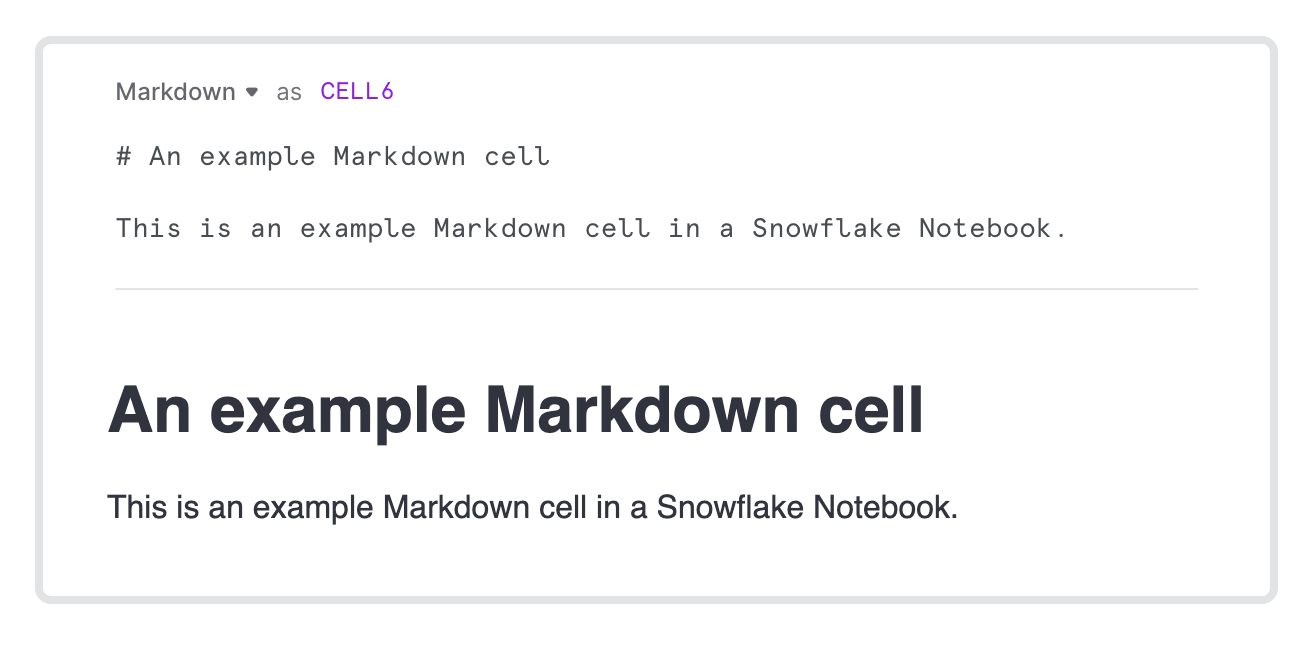
To view only the formatted text, select another cell. When the Markdown cell is no longer selected, only the rendered Markdown is visible.
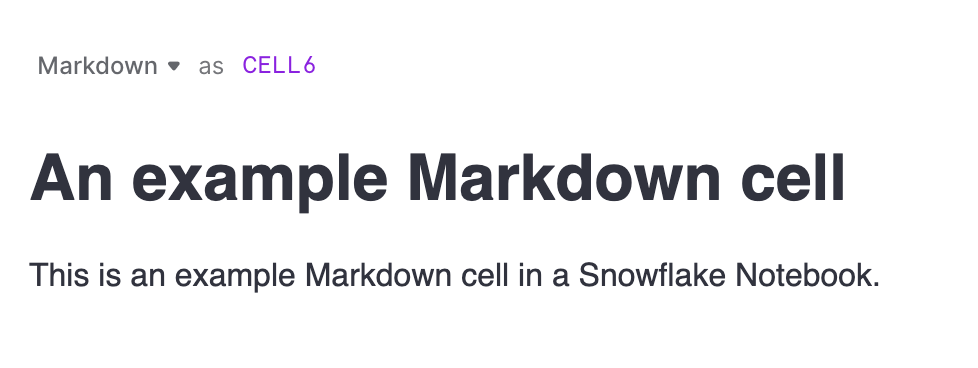
Visualize results of cells by using Streamlit¶
You can visualize the results of cells using one of the following libraries:
Visualize results by using Streamlit¶
You can use any chart elements supported by Streamlit version 1.22.0 to create a line chart, bar chart, area chart, scatter plot, or a map with points on it. See Chart elements in the API reference of the Streamlit library documentation.
참고
Some Streamlit chart elements are not supported in Snowflake or might be subject to additional terms. See Considerations for writing notebooks and adding cells.
For example, to reference a SQL cell CELL1
that outputs two columns of data as a table, where CELL1
includes the following SQL code:
SELECT 'FRIDAY' as SNOWDAY, 0.2 as CHANCE_OF_SNOW
UNION ALL
SELECT 'SATURDAY',0.5
UNION ALL
SELECT 'SUNDAY', 0.9;
To visualize the results in a line chart, you can use the following Python code to reference the results of the SQL cell, convert it to a Snowpark DataFrame and then a Pandas DataFrame, then display a line chart:
import streamlit as st
my_df = cells.CELL1.to_df().to_pandas()
st.line_chart(my_df, x='SNOWDAY', y='CHANCE_OF_SNOW')
After you run both cells, the following visualization appears:
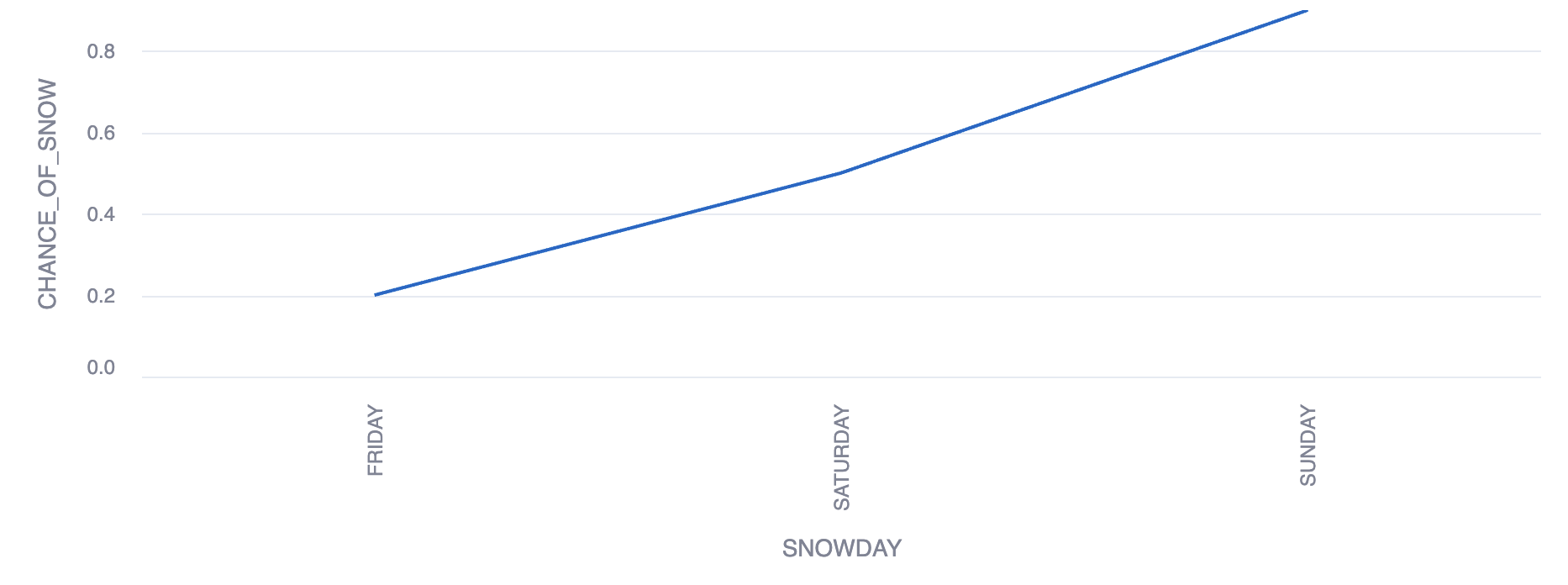
For details on referencing other cells, see Reference results and chain cells in a notebook.
Visualize results by using Altair¶
To visualize results using Altair visualizations, use the Streamlit st.altair_chart
element.
For details on the Altair chart element, see st.altair_chart.
For details on available visualization types when using Altair, see Vega-Altair: Declarative Visualization in Python.
Snowflake Notebooks currently support Altair version 4.0.
For example, to display a heat map based on the output of SQL cell CELL3
, where CELL3
includes the following SQL code:
SELECT 1 as "a", 1 as "b", 35 as "c"
UNION ALL
SELECT 17, 7, 2
UNION ALL
SELECT 14, 29, 18;
Write the following Python code in a Python cell to reference the output of the SQL cell, process the output into a Snowpark DataFrame and then a Pandas DataFrame, create an Altair chart object, and then use Streamlit to display the chart:
import streamlit as st
import altair as alt
my_df = cells.CELL3.to_df().to_pandas()
chart = (
alt.Chart(my_df)
.mark_rect()
.encode(x="a", y="b", color="c")
)
st.altair_chart(chart, use_container_width=False, theme=None)
After you run both cells, the following visualization appears:
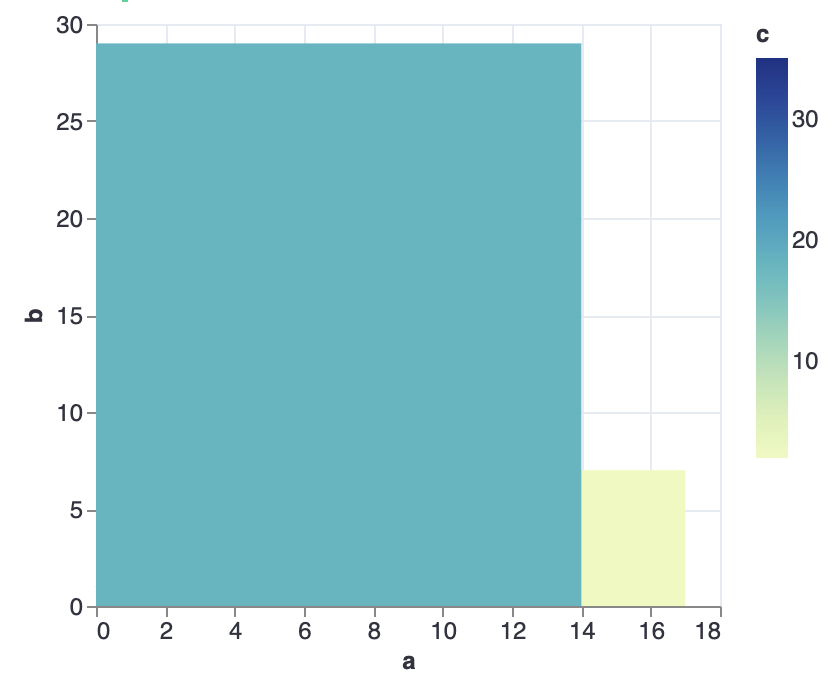
For details on referencing other cells, see Reference results and chain cells in a notebook.
Visualize results with Matplotlib¶
To visualize results using Matplotlib visualizations, use the Streamlit st.pyplot
element.
To use a Matplotlib visualization, install the Matplotlib library for your notebook:
From the notebook, select Packages.
Locate the matplotlib library and select the library to install it.
Write code to visualize your results.
For example, to visualize a bar chart of a random array of numbers, add the following code to a Python cell:
import streamlit as st
import matplotlib.pyplot as plt
import numpy as np
arr = np.random.normal(1, 1, size=100)
fig, ax = plt.subplots()
ax.hist(arr, bins=20)
st.pyplot(fig)
After you run the cell, the following visualization appears:
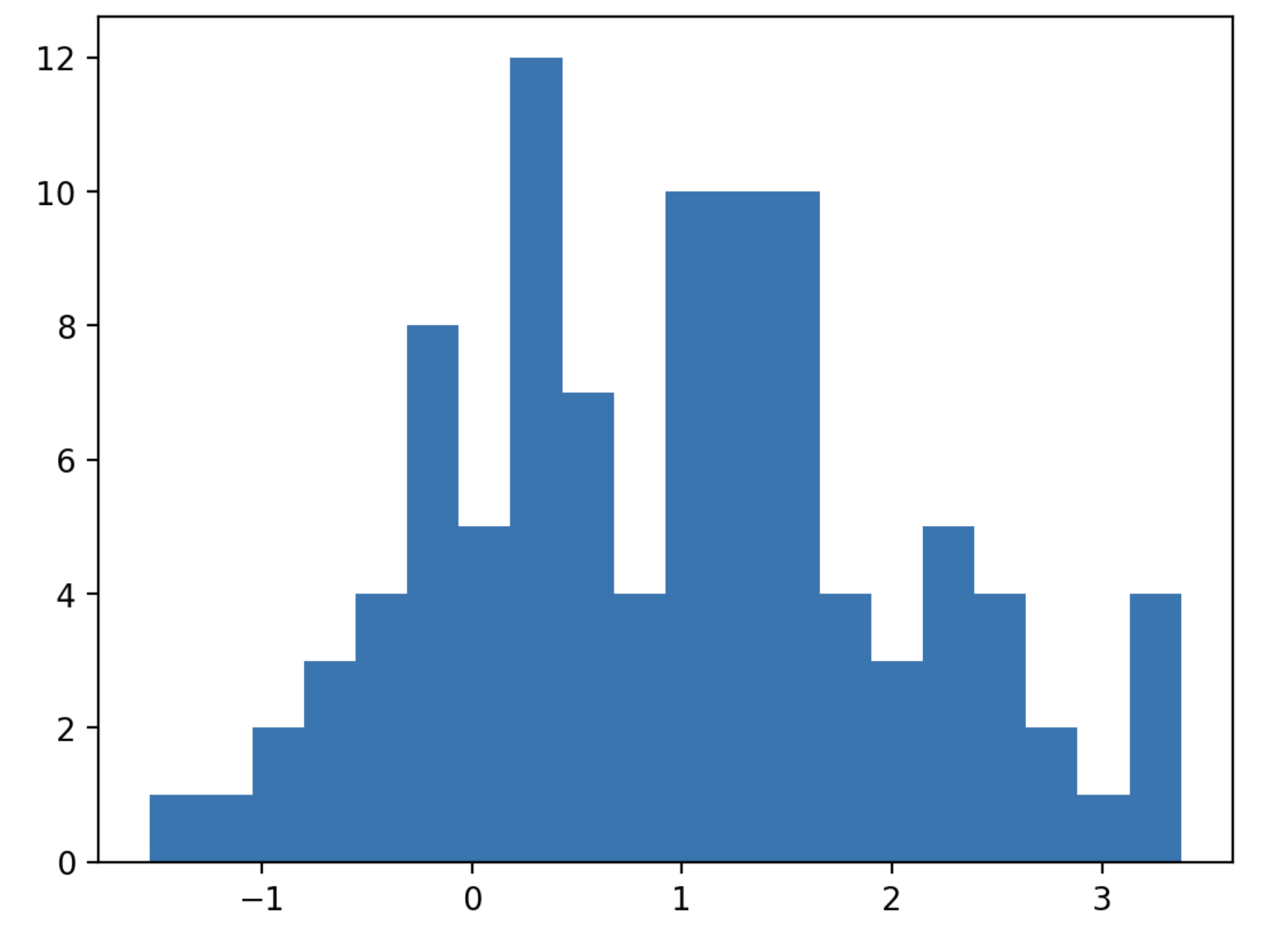
For more details on using the st.pyplot
chart element, see
st.pyplot.
참고
Visualizations created with this library are not cached.
Visualize results with seaborn¶
To visualize results using seaborn visualizations, use the Streamlit st.pyplot
element in combination with the
seaborn library.
To use a seaborn visualization, you must install both the seaborn library and the Matplotlib library for your notebook:
From the notebook, select Packages.
Locate the seaborn library and select the library to install it.
Locate the matplotlib library and select the library to install it.
Write code to visualize your results.
To use seaborn, use the st.pyplot
, but instead of passing a Matplotlib figure
into the fig
parameter, pass a seaborn figure.
For example, to display a bar chart based on the output of SQL cell CELL0
, where CELL0
includes the following SQL code:
SELECT 1 as "a", 1 as "b", 35 as "c"
UNION ALL
SELECT 17, 7, 2
UNION ALL
SELECT 14, 29, 18;
Write the following Python code in a Python cell:
import streamlit as st
import seaborn as sns
import matplotlib.pyplot as plt
f, ax = plt.subplots(figsize=(3, 3))
fig=f.figure
my_df = cells.CELL0.to_df().to_pandas()
sns.set_color_codes("pastel")
sns.barplot(x="a", y="b", data=my_df,
label="Total", color="c")
st.pyplot(fig)
After you run both cells, the following visualization appears:
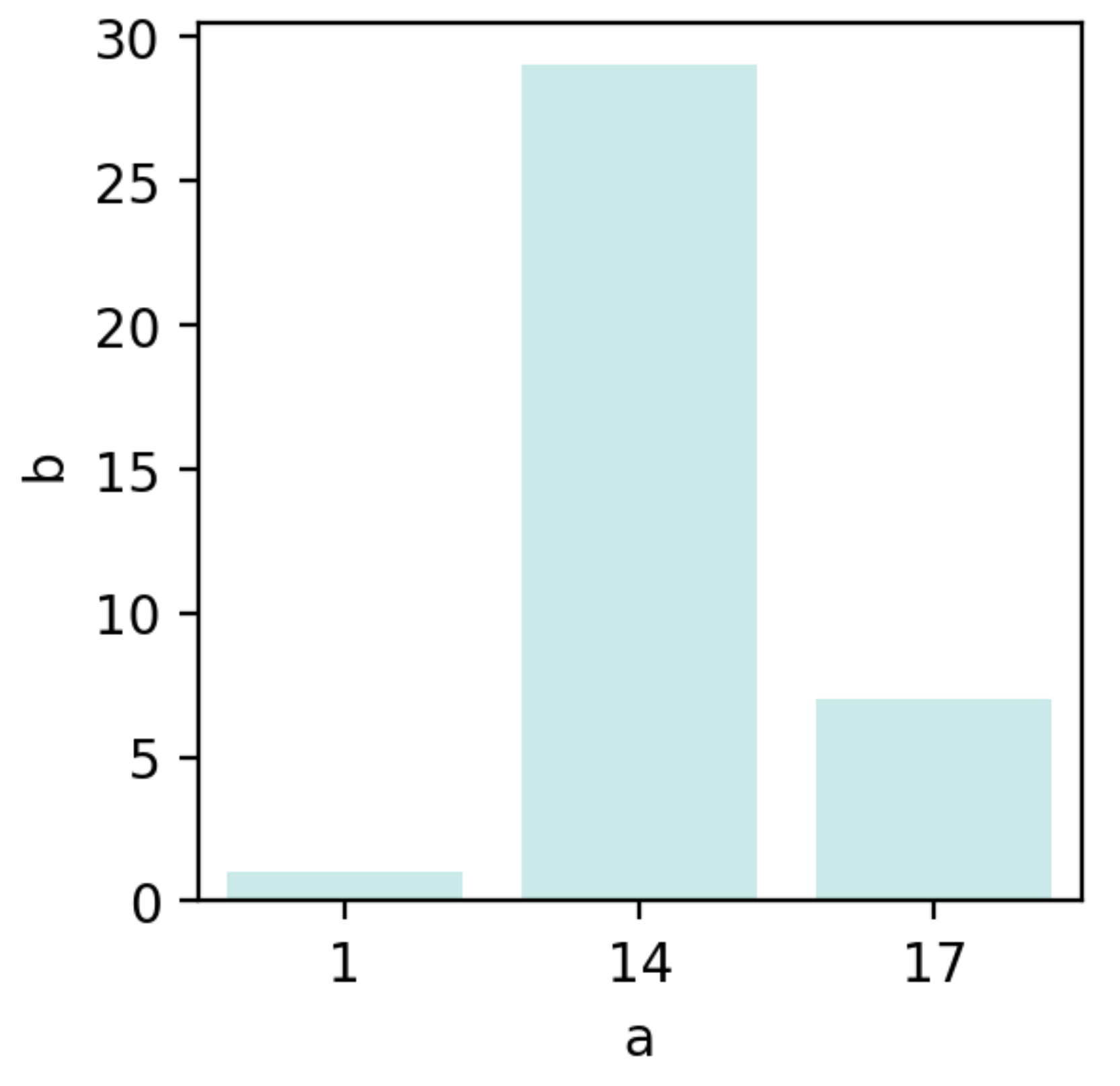
For more details on using the st.pyplot
chart element, see
st.pyplot.
For examples of seaborn visualizations, see the seaborn Example gallery.
참고
Visualizations created with this library are not cached.
Reference results and chain cells in a notebook¶
You can reference the results of previous cells in a cell in your notebook.
For example, if you want to filter or visualize a table produced by a SQL or Python cell, refer to the following table:
Reference cell type |
Current cell type |
Reference syntax |
Example |
---|---|---|---|
SQL |
Python |
|
The cell name of the reference is case-sensitive, and you must include the notation to convert the SQL table of results to a Snowpark DataFrame. st.line_chart(cells.CELL1.to_df())
|
SQL |
SQL |
|
The cell name of the reference is case-sensitive and must exactly match the name of the referenced cell. SELECT * FROM {{cells.CELL2}} where PRICE > 500
|
Python |
SQL |
|
For example, in a Python cell named d = {'col1': [1, 2], 'col2': [3, 4]}
You can reference the value of the variable SELECT * FROM my_table WHERE COUNTRY = {{d}}
|
Python |
Python |
|
Reference results from another Python cell as a variable. The cell name of the reference is case-sensitive and must exactly match the name of the referenced cell. It references the return value of the last statement in the referenced cell. For example, in a Python cell named d = {'col1': [1, 2], 'col2': [3, 4]}
If the variable is called in the Python cell, you can reference the value of the variable st.line_chart(cells.CELL1)
You can also reference the variable directly: st.line_chart(d)
|
Run code and SQL in Snowflake Notebooks¶
To run code and SQL commands in a Snowflake Notebook, you can:
Run a single cell: Choose this option when making frequent code updates.
Run all cells in a notebook in sequential order: Choose this option before presenting or sharing a notebook to ensure that the recipients see the most current information.
Press CMD + Shift + Return on a Mac keyboard, or CTRL + Shift + Enter on a Windows keyboard.
Select Run all.
Run a cell and advance to the next cell: Choose this option to run a cell and move on to the next cell more quickly.
Press Shift + Return on a Mac keyboard, or Shift + Enter on a Windows keyboard.
Select the vertical ellipsis (more actions) for a cell, and choose Run cell and advance.
Run all above: Choose this option when running a cell that references the results of earlier cells.
Select the vertical ellipsis (more actions) for a cell, and choose Run all above.
Run all below: Choose this option when running a cell that later cells depend on. This option runs the current cell and all following cells.
Select the vertical ellipsis (more actions) for a cell, and choose Run all below.
Warehouse recommendations for running Snowflake Notebooks¶
When you create a notebook, you select a warehouse in which to run the Snowflake Notebook processes and any code. Snowflake recommends using an X-Small warehouse to minimize credit consumption.
You might want to run large queries using SQL or perform compute-intensive operations using Snowpark Python that require a larger warehouse. In this case, you have two options:
Specify a warehouse to use for a specific cell or set of cells.
This ensures that the queries in those cells use the larger warehouse, but notebook processes continue to run on the smaller notebook warehouse, so you’ll use less compute when you’re not running queries.
For example, to specify a warehouse called
notebooks
to use for specific cells, you can add a SQL cell with the USE WAREHOUSE command or add the command to the Python code in a Python cell:- SQL::
USE WAREHOUSE notebooks;
- Python::
session.sql('USE WAREHOUSE notebooks;').collect()
After you run any cell specifying
USE WAREHOUSE
, all cells that run in the remainder of the notebook session use the specified warehouse. If you no longer need queries to run with a large warehouse, write a new cell to specify a smaller warehouse to use.Choose a larger warehouse for the entire notebook.
If you choose this option, all queries that run in the notebook have more compute available, but the warehouse runs for the entire notebook session, even if queries do not run.
조심
Choosing this option can dramatically increase credit consumption for the entire notebook.
To change the notebook warehouse:
Select the notebook title, and then select Notebook settings.
Select a new warehouse to use.
Keyboard shortcuts for notebooks¶
Snowflake Notebooks support various keyboard shortcuts to help accelerate your development process.
Task |
MacOS |
Windows |
---|---|---|
Open the list of keyboard shortcuts. |
? |
? |
Run all cells. |
CMD + Shift + Return |
CTRL + Shift + Enter |
Run the selected cell. |
CMD + Return |
CTRL + Enter |
Run the selected cell and advance to the next cell. |
Shift + Return |
Shift + Enter |
Move between cells. |
Up and down arrows |
Up and down arrows |
Find within the cell. |
CMD + f |
CTRL + f |
Add a cell above the currently selected cell. |
a |
a |
Add a cell below the currently selected cell. |
b |
b |
Delete the currently selected cell. |
dd |
dd |
Convert a SQL or Python cell into a Markdown cell. |
m |
m |
Convert a cell into a code cell:
|
y |
y |
In addition, you can use the same keyboard shortcuts that you use for worksheets. See 바로 가기 키로 워크시트 업데이트하기.